Machine Learning Visualization
Worked in a team of five to create a 3D visualization tool for machine learning algorithms as part of a capstone project for a professor at ASU. This tool will be incorporated into the professor’s course.
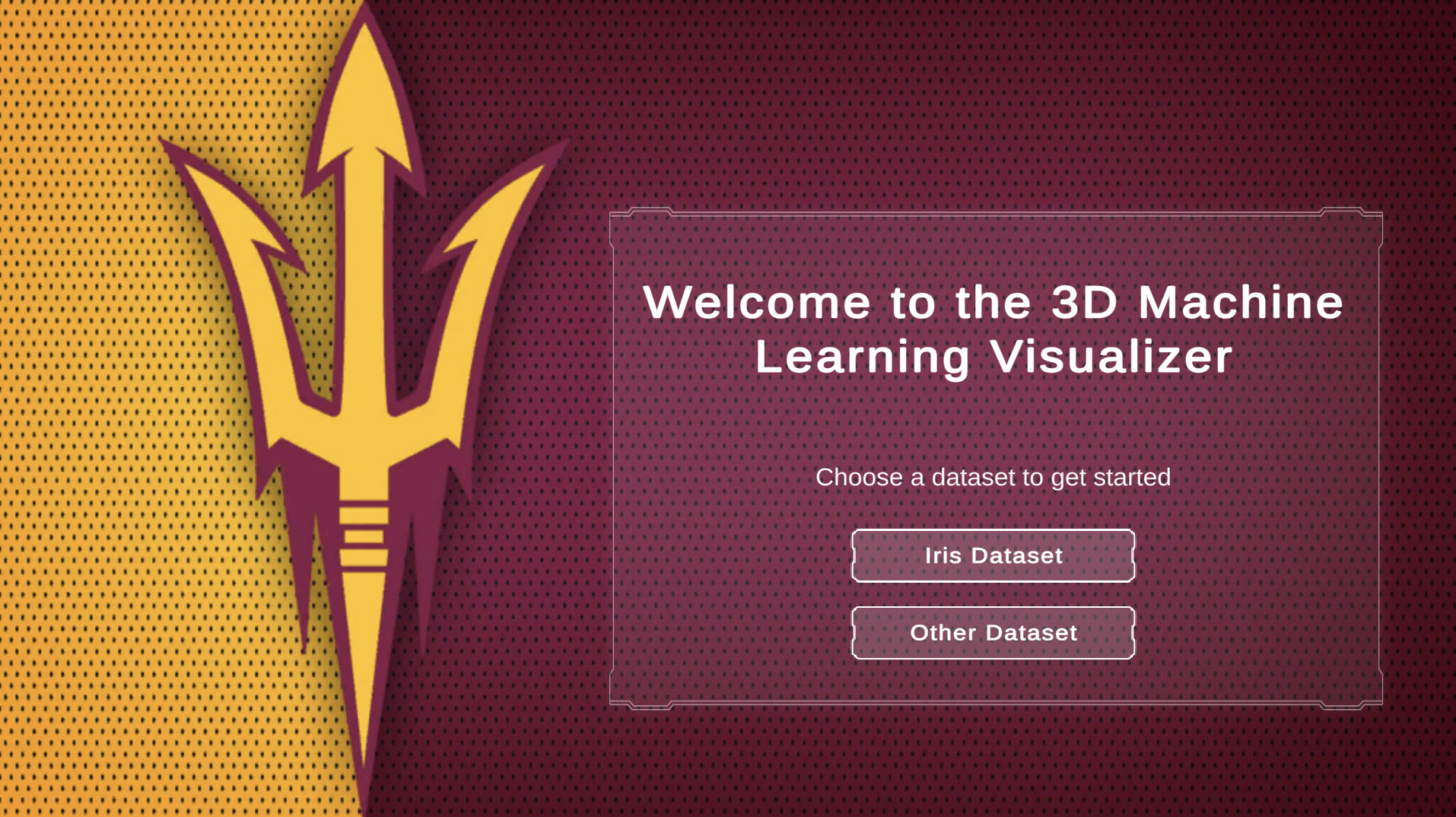
In this snippet, data points from the Iris dataset are instantiated in Unity. Each data point is positioned in 3D space based on sepal and petal dimensions. The points are assigned sizes and colors to represent the different species of Iris flowers, enhancing the visualization of machine learning classifications.
// Instantiating Data Points in Unity
foreach (DataPoint point in dataPoints) {
float scaler = 20.0f;
Vector3 position = new Vector3(
point.sepal_length * scaler + 720 - xMin * scaler,
point.sepal_width * scaler + 547 - yMin * scaler,
-point.petal_length * scaler + 56 + zMin * scaler
);
GameObject sphere = Instantiate(dataPointPrefab, position, Quaternion.Euler(-90, 0, 0));
float scale = 20.0f + (point.petal_width * 10.0f);
sphere.transform.localScale = new Vector3(scale, scale, scale);
Material chosenMaterial = point.prediction == "Iris-setosa" ? SetosaMaterial : NonSetosaMaterial;
sphere.GetComponent().material = chosenMaterial;
}
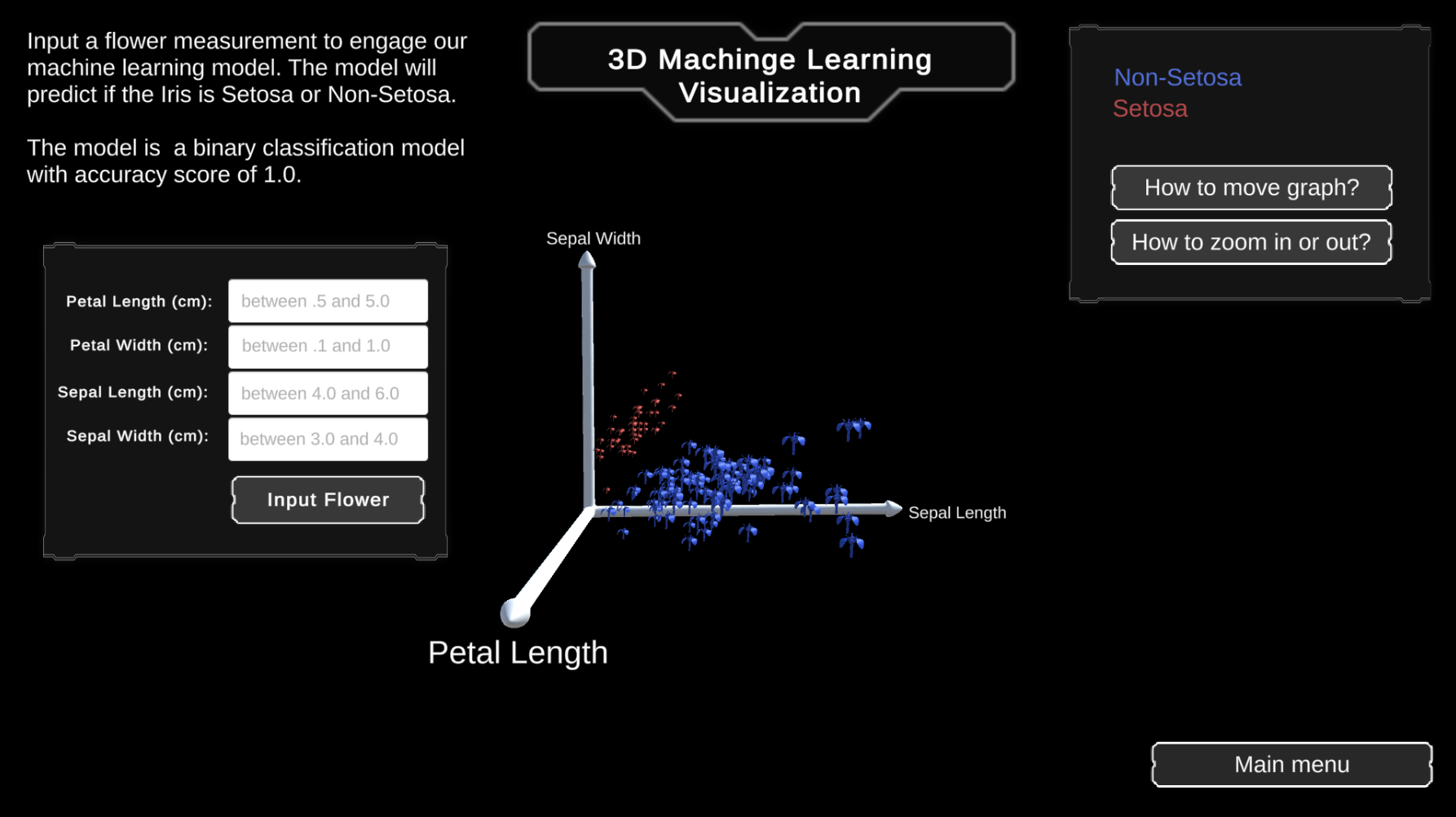
This function is responsible for generating real-time predictions based on user input. It uses a trained model to classify new Iris flower data, allowing for immediate feedback, which helps the user interact with the visualization in an engaging way.
// Predicting Based on User Input
private string Predict(float[] input) {
client.Predict(input, output => {
prediction = "Prediction: " + output;
}, error => {
// Handle error
});
return prediction;
}