3D Lunar Base Exploration
Designed a 3D environment with a moon base, custom terrain, skybox, and animations, including a third-person controller. I built the environment using Unity and C#.
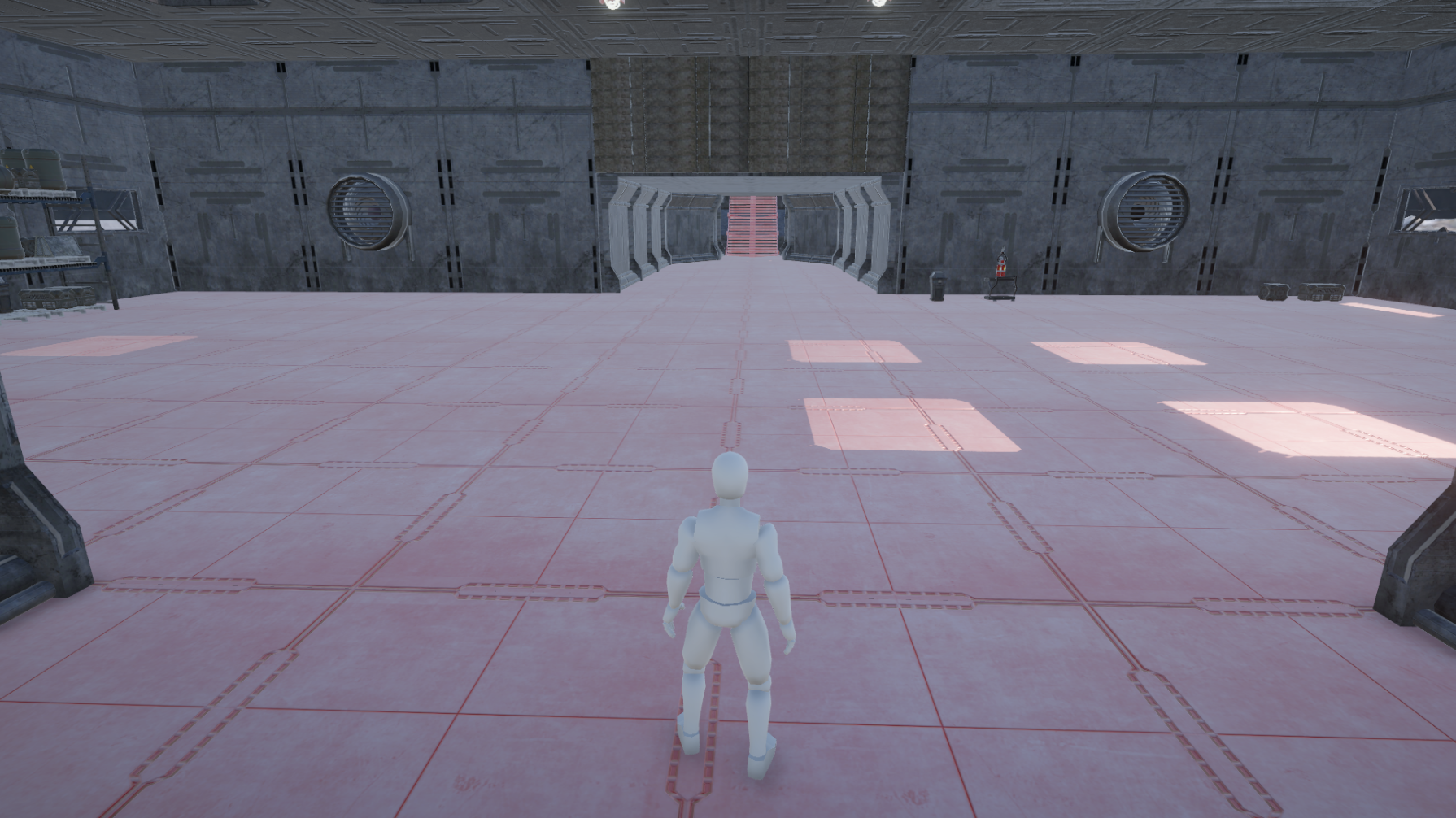
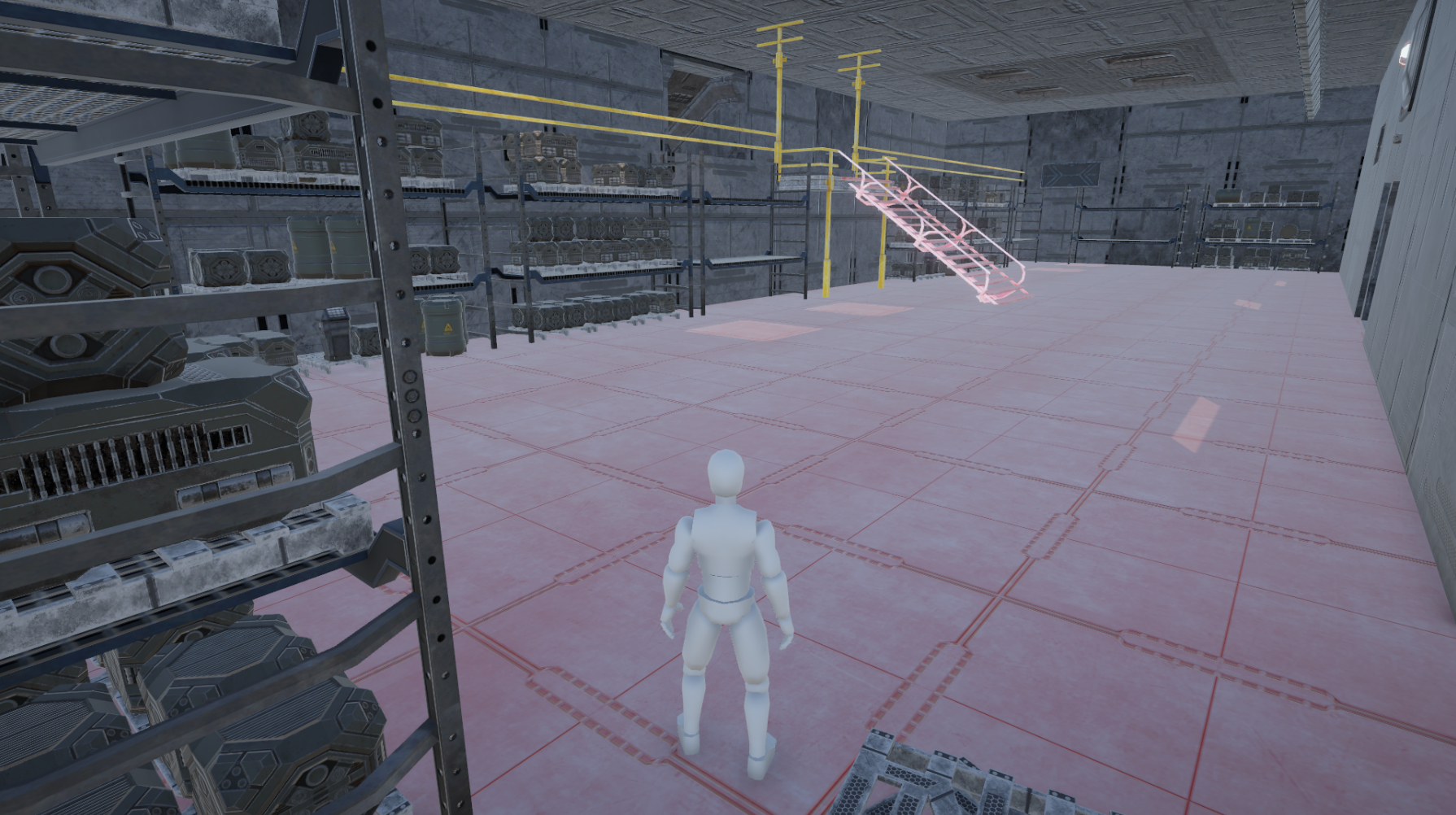
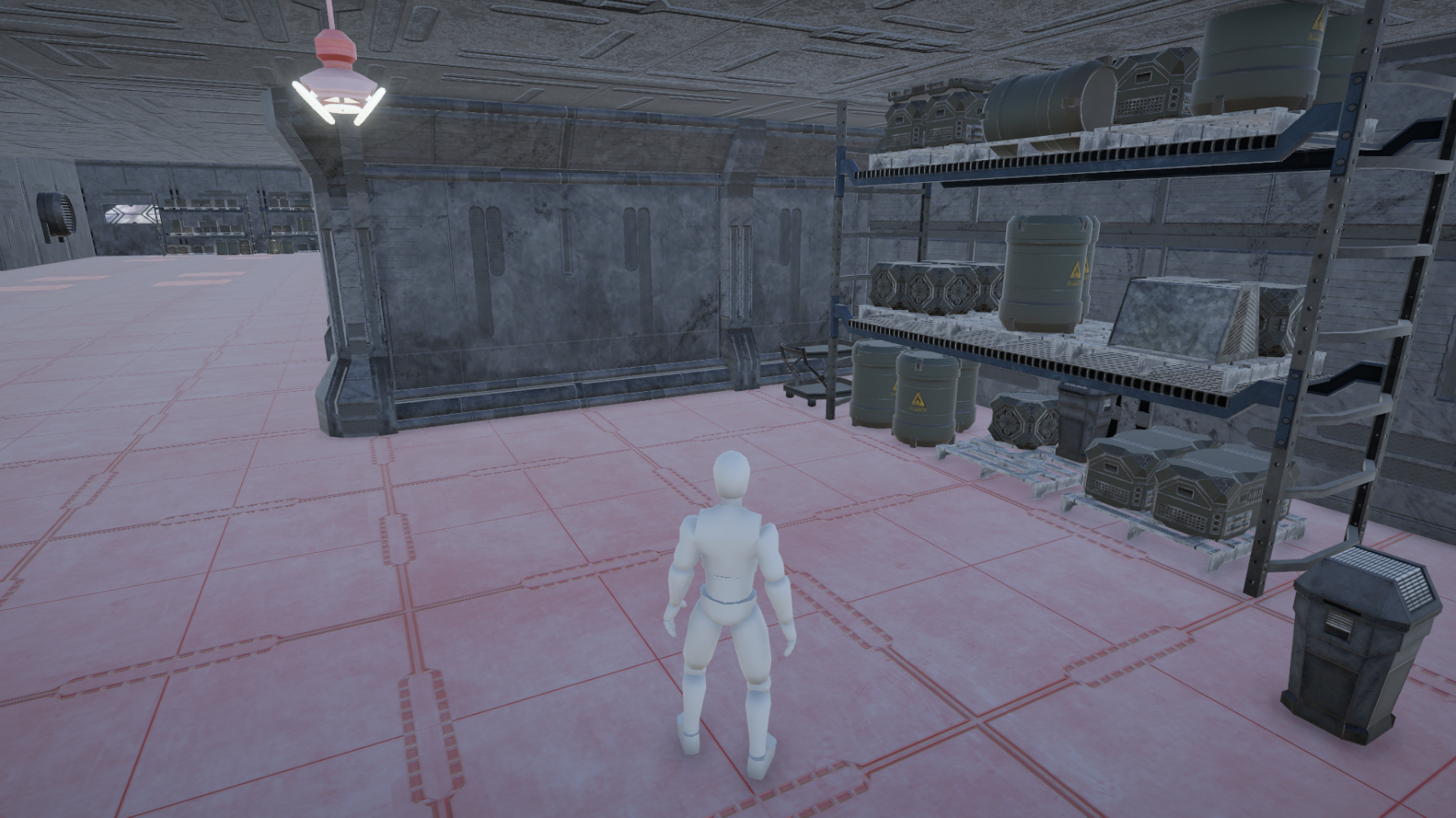
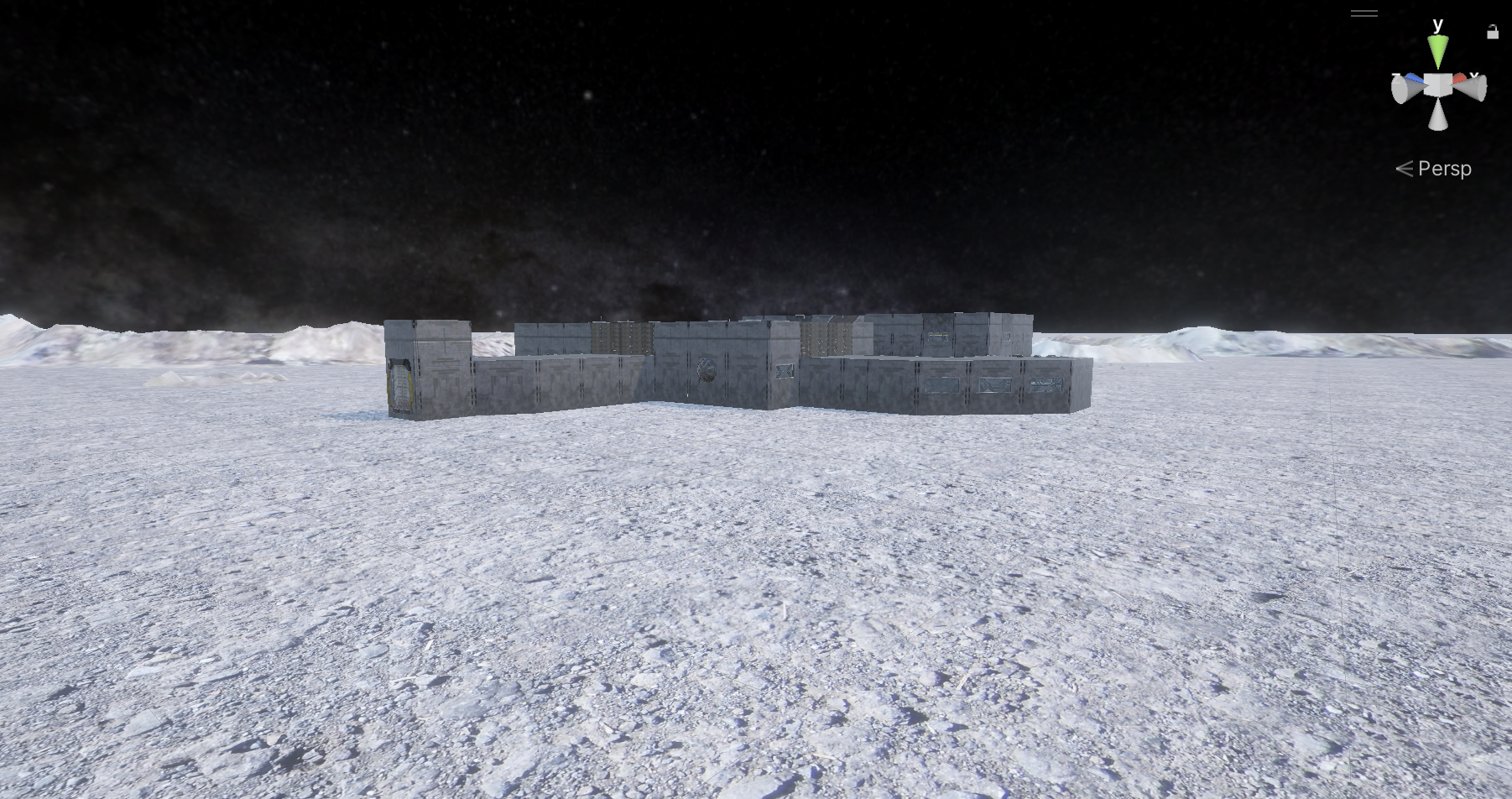
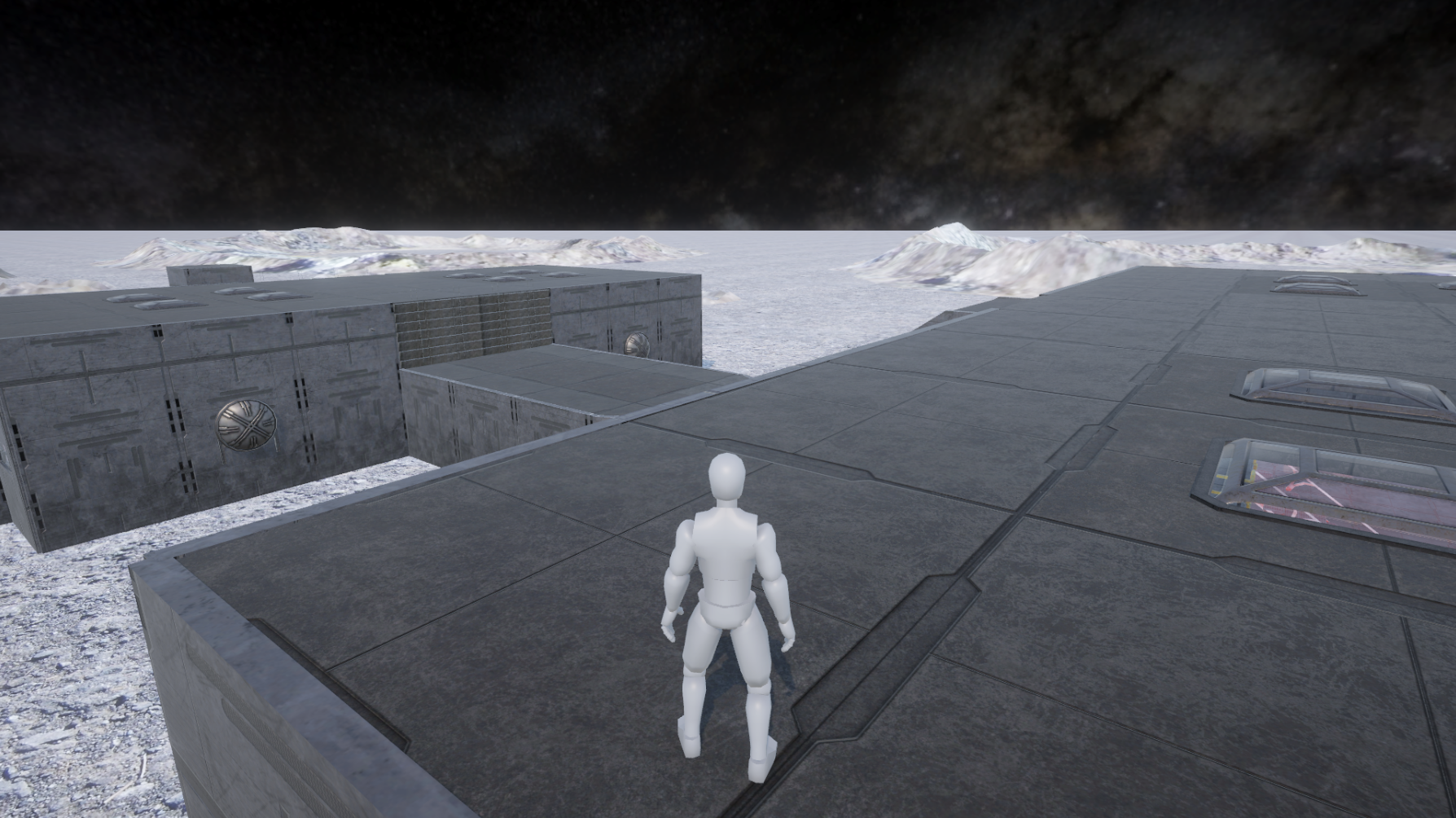
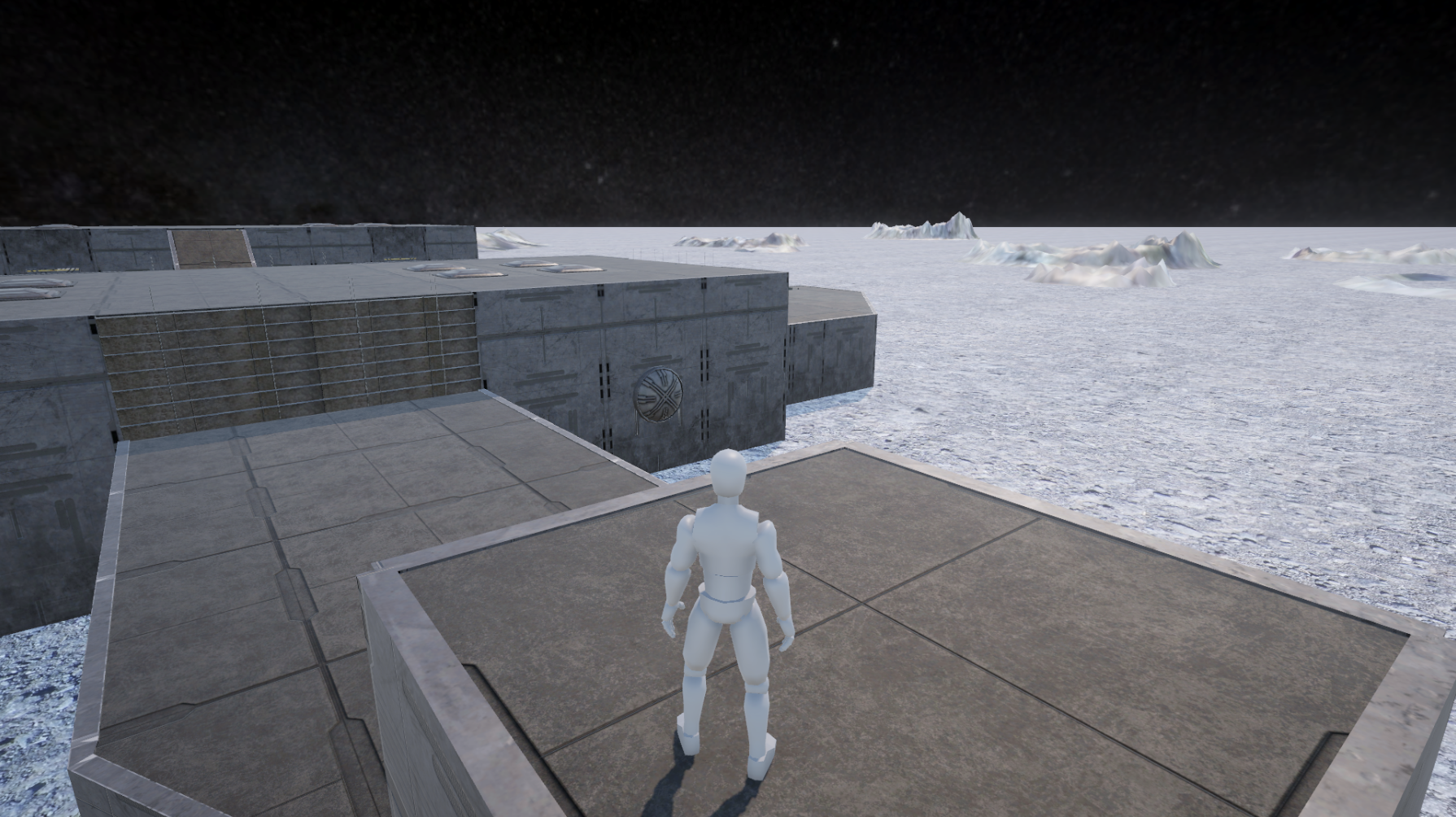
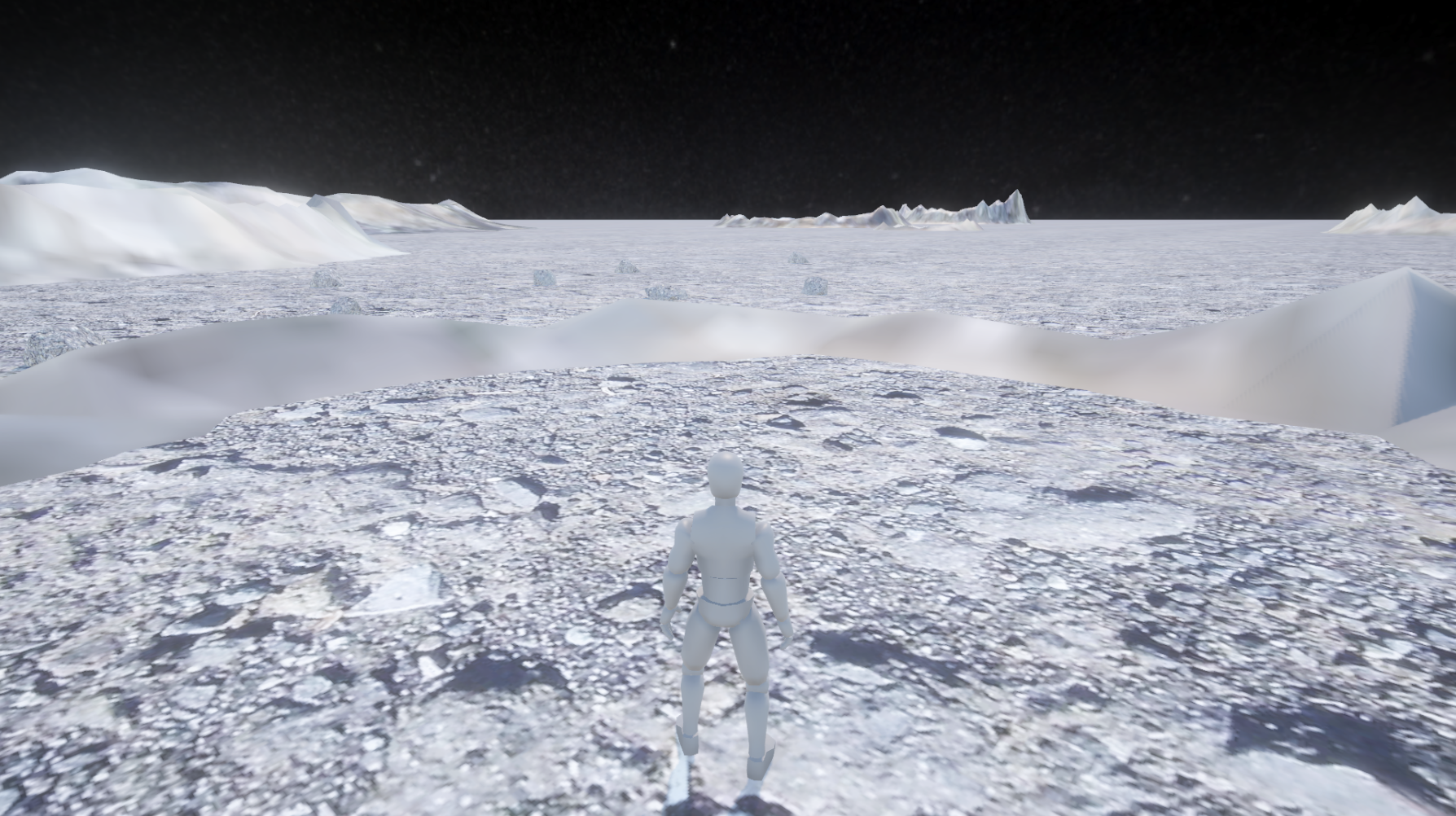
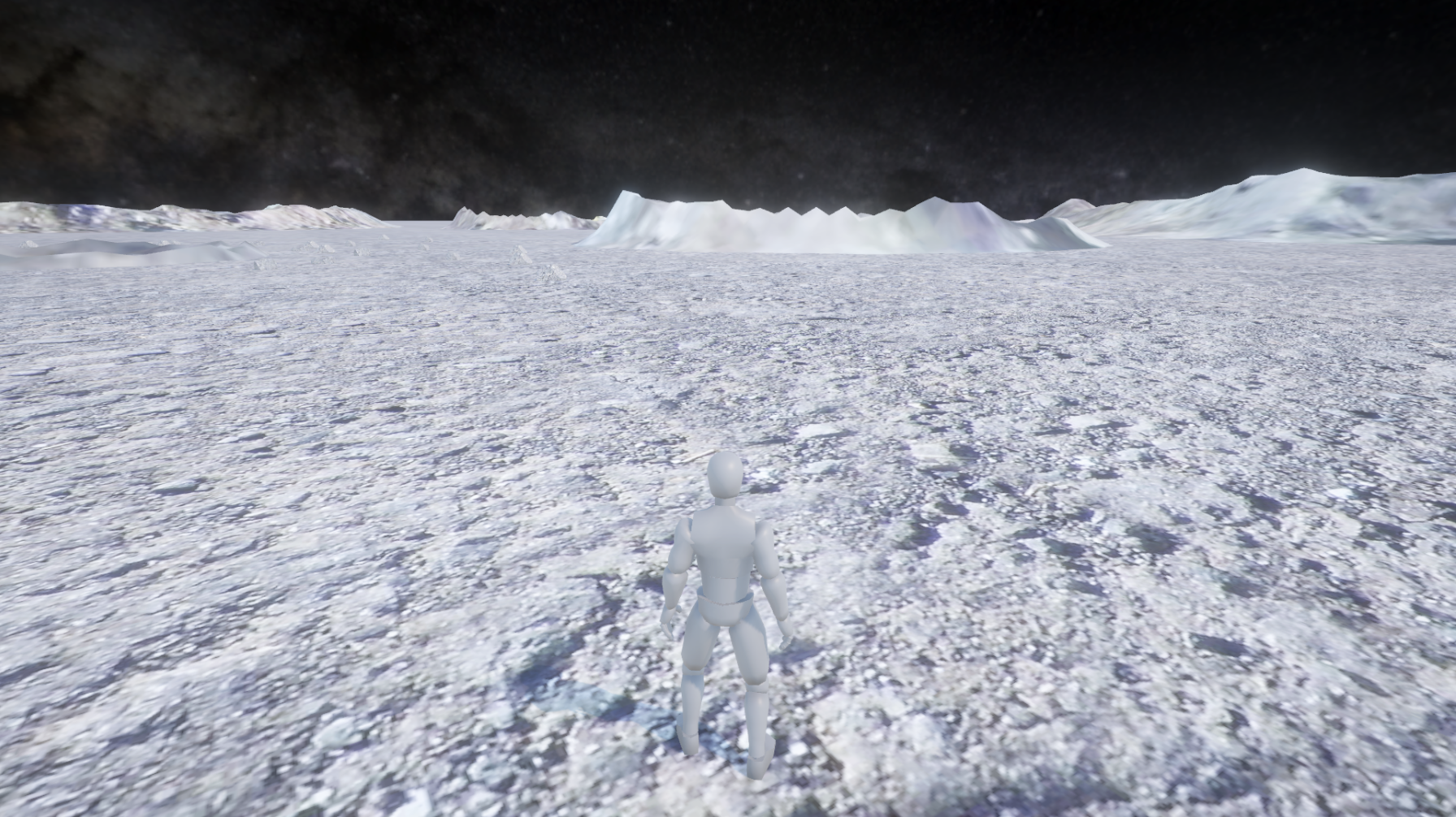
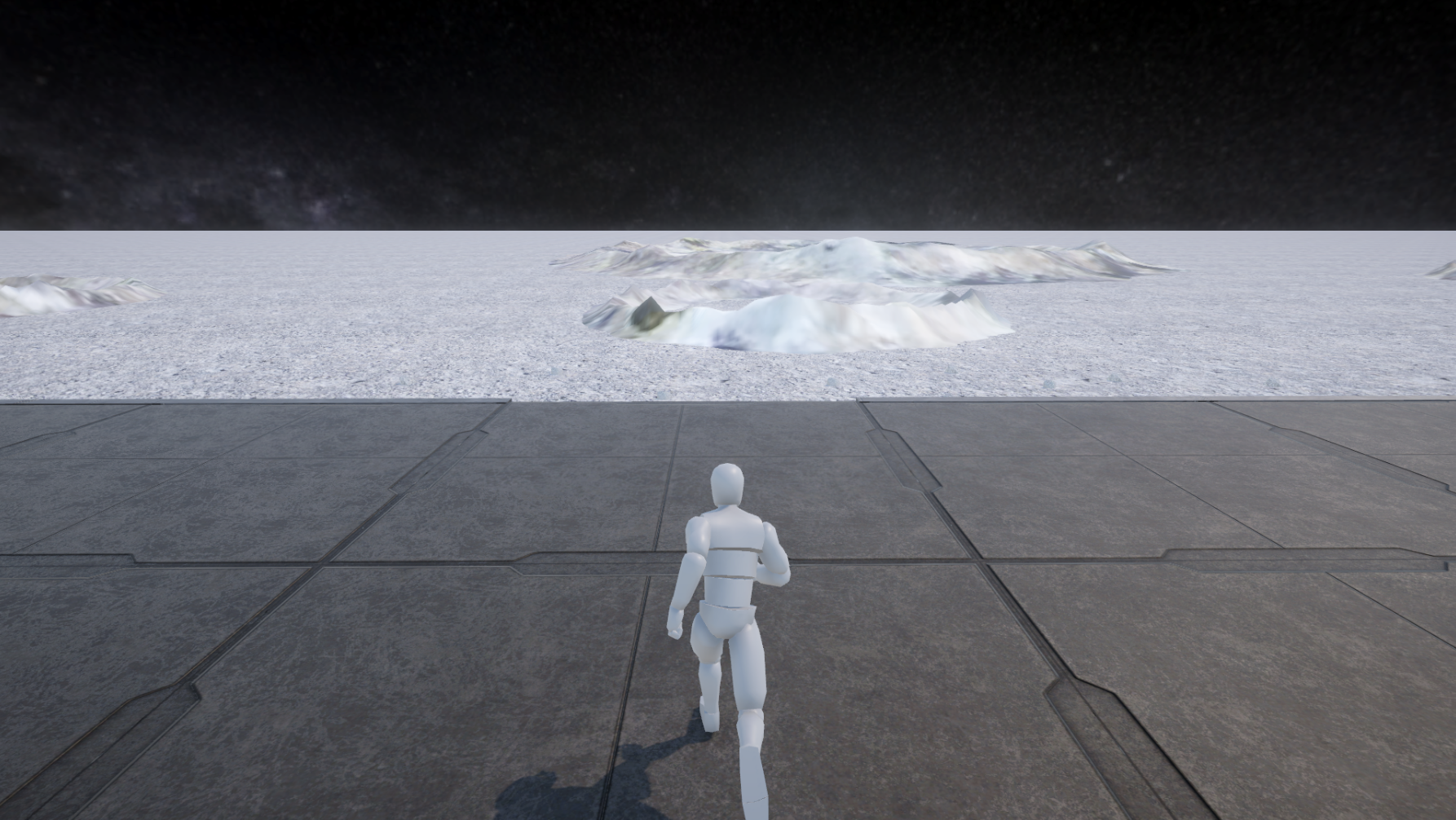
The following state diagram is used to control which animations are played for the 3rd person controller:
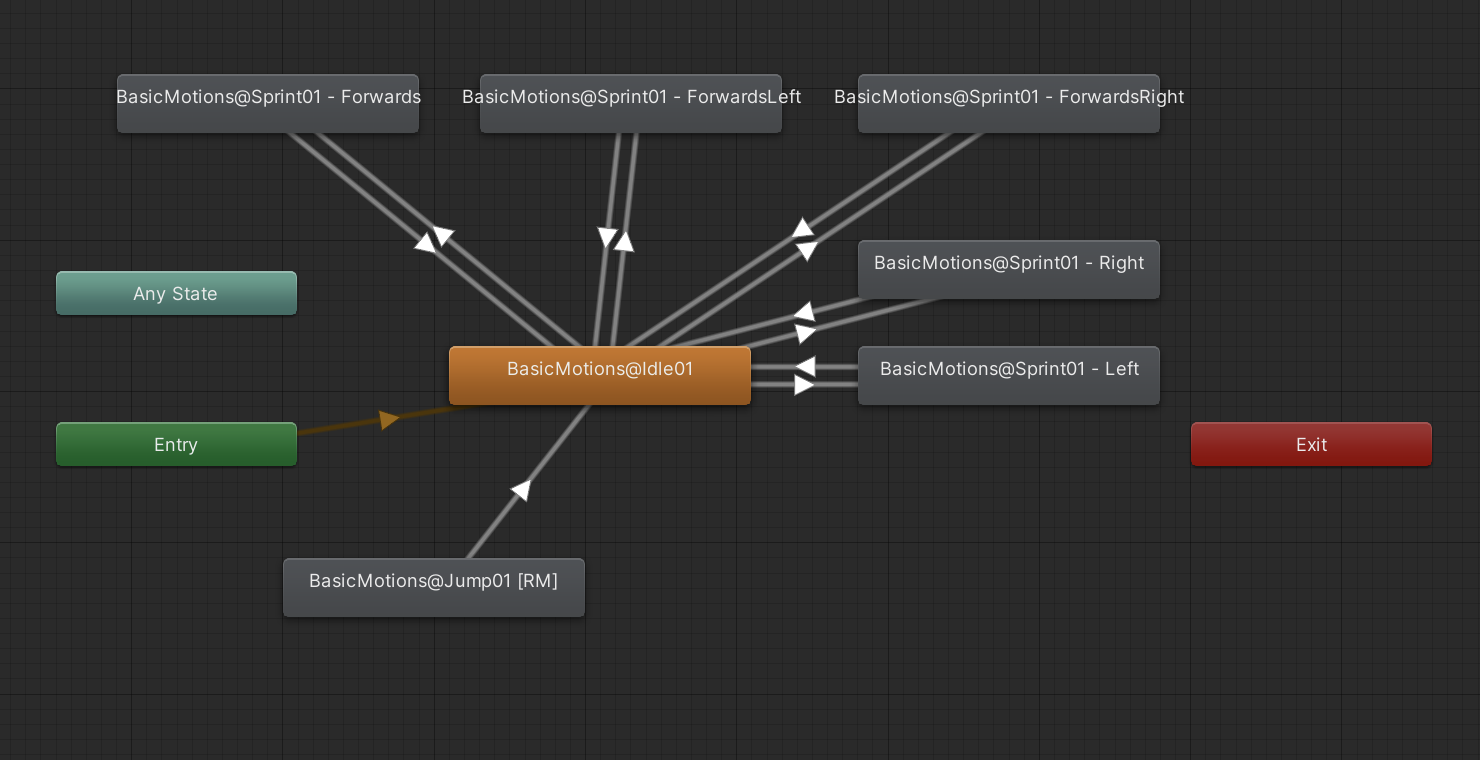
The following function is how the state diagram above for animations is called in the code:
public void OnMove(InputAction.CallbackContext context) {
moveVector = context.ReadValue();
animator.SetBool("isSprinting", false);
animator.SetBool("isSprintingHardLeft", false);
animator.SetBool("isSprintingLeft", false);
animator.SetBool("isSprintingHardRight", false);
animator.SetBool("isSprintingRight", false);
if (moveVector.magnitude > 0) {
if (moveVector.x < 0 && moveVector.y == 0) {
// Only left (A key pressed)
animator.SetBool("isSprintingHardLeft", true);
}
else if (moveVector.x < 0 && moveVector.y > 0) {
// Forward left (W and A pressed)
animator.SetBool("isSprintingLeft", true);
}
else if (moveVector.x > 0 && moveVector.y == 0) {
// Only right (D key pressed)
animator.SetBool("isSprintingHardRight", true);
}
else if (moveVector.x > 0 && moveVector.y > 0) {
// Forward right (W and D pressed)
animator.SetBool("isSprintingRight", true);
}
else if (moveVector.x == 0 && moveVector.y > 0) {
// Only forward (W key pressed)
animator.SetBool("isSprinting", true);
}
}
else {
animator.SetBool("isSprinting", false);
}
}